OpenCV (4) Image and Video
1. Read and write a image:
Read a image and convert it to a gray-scale image. Save the gray-scale image.
#include<iostream> #include<opencv2/opencv.hpp> using namespace std; using namespace cv; int main() { Mat gray_img = imread("data/image/mini.jpg", IMREAD_GRAYSCALE); imshow("mini", gray_img); waitKey(0); destroyAllWindows(); // save the image. imwrite("data/image/gray_mini.jpg", gray_img); return 0; }
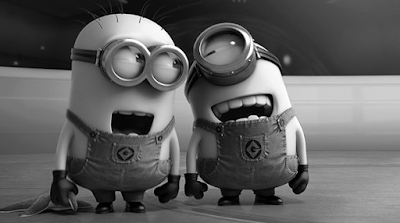
2. Open a video:
The video is composed of a series of graphics, each one is called frame.
#include<iostream> #include<opencv2/opencv.hpp> using namespace std; using namespace cv; int main() { // init an object of VideoCapture. VideoCapture cap("data/video/ggo.mp4"); // make sure that the video is opened successfully. if (!cap.isOpened()) { cout << "Capture open fail !!" << endl; } Mat frame; // read the video frame by frame. // if the frame is not a null frame, display the frame. while(cap.read(frame)) { imshow("frame", frame); // break the while loop if user press esc. char key = (char) waitKey(30); if (key == 27) { break; } } destroyAllWindows(); return 0; }
3. cv::VideoCapture::operator>>():
cv::VideoCapture::operator>>() is similar to std::istream::operator>>().
Mat frame; cap >> frame; while(!frame.empty()) { imshow("frame", frame); char key = (char) waitKey(30); if (key == 27) { break; } cap >> frame; }
4. Use VideoCapture to get the information of the video:
int main() { VideoCapture cap("data/video/prince.mp4"); if (!cap.isOpened()) { cout << "Capture open fail !!" << endl; } cout << "width: " << cap.get(CAP_PROP_FRAME_WIDTH) << endl; cout << "height: " << cap.get(CAP_PROP_FRAME_HEIGHT) << endl; cout << "FPS: " << cap.get(CAP_PROP_FPS) << endl; return 0; }
Reference
[1] Adrian Kaehler, Gary Bradski, Learning OpenCV 3 , O'Reilly Media (2017)