OpenCV (3) OpenCV Drawing and Annotating
1. Draw a circle:
OpenCV provides some functions to make lines, squares, circles on the top of an image.
For example, we can draw a circle on our image.
#include#include using namespace std; using namespace cv; int main() { Mat img = imread("mini.jpg"); circle( img, // draw on the top of this image Point(100, 60), // center 60, // radius Scalar(0x00, 0x00, 0xFF), // color (BGR) 2 // thickness ); imshow("Image", img); waitKey(0); destroyAllWindows(); return 0; }
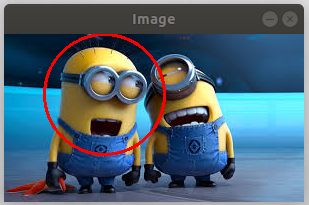
2. Annotation:
Furthermore, we can put some text on our image.
#include#include using namespace std; using namespace cv; int main() { Mat img = imread("mini.jpg"); circle( img, Point(100, 60), // center 60, // radius Scalar(0x00, 0x00, 0xFF), // color (BGR) 2 // thickness ); putText( img, String("minion A"), // annotation Point(10, 30), // position FONT_HERSHEY_PLAIN, // font 1, // font size Scalar(0x00, 0x00, 0xFF) // color (BGR) ); imshow("Image", img); waitKey(0); destroyAllWindows(); return 0; }
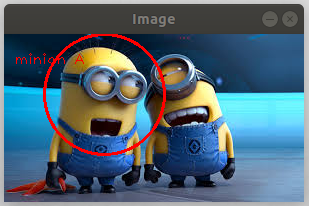
Reference
[1] Adrian Kaehler, Gary Bradski, Learning OpenCV 3 , O'Reilly Media (2017)